Interactive Components with CSS
Build navigation bars, dropdown menus, and image galleries with modern CSS techniques
Learning Outcomes
- Create horizontal and vertical navigation bars with hover effects
- Implement dropdown menus that appear on hover or click
- Build responsive image galleries using CSS Grid and Flexbox
- Add interactive elements and transitions to enhance user experience
- Apply accessibility best practices to interactive components
Dropdown Menus
Dropdown menus reveal additional navigation options when users hover or click on a parent item.
Mega Menu
<div class="dropdown">
<button class="dropdown-btn">Menu <i class="fa fa-caret-down"></i></button>
<div class="dropdown-content">
<a href="#"><i class="fa fa-icon"></i> Item 1</a>
<a href="#"><i class="fa fa-icon"></i> Item 2</a>
</div>
</div>
<style>
.dropdown {
position: relative;
display: inline-block;
}
.dropdown-content {
display: none;
position: absolute;
background-color: white;
min-width: 200px;
box-shadow: 0 8px 16px rgba(0,0,0,0.2);
z-index: 1;
}
.dropdown:hover .dropdown-content {
display: block;
}
.dropdown-content a {
color: var(--text-color);
padding: 12px 16px;
display: block;
transition: background-color 0.3s;
}
.dropdown-content a:hover {
background-color: var(--burgundy-lighter);
}
</style>
Image Galleries
Modern CSS provides powerful tools like Flexbox and Grid to create responsive image galleries.
Flexbox Gallery
A flexible gallery that adjusts to different screen sizes using Flexbox.
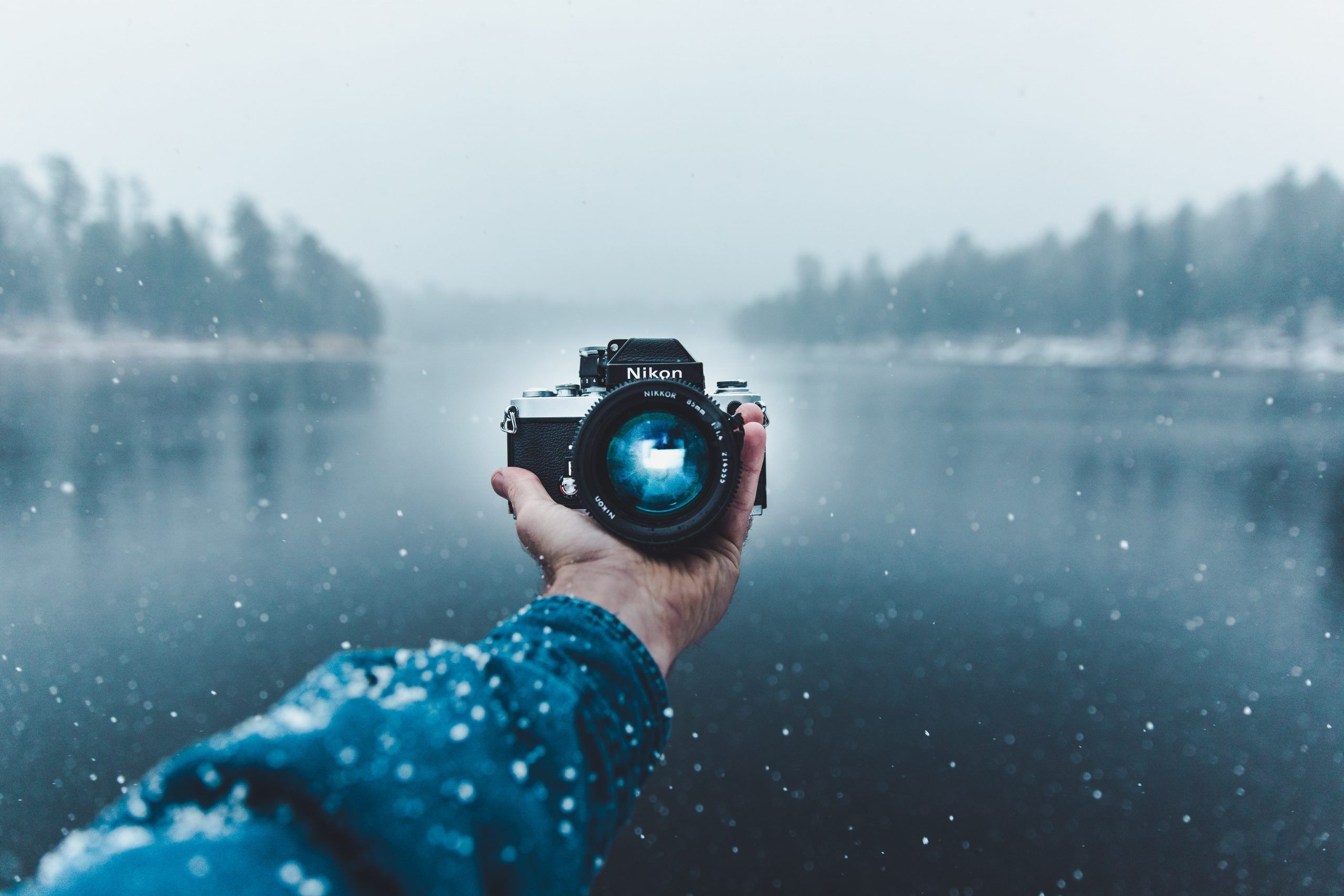
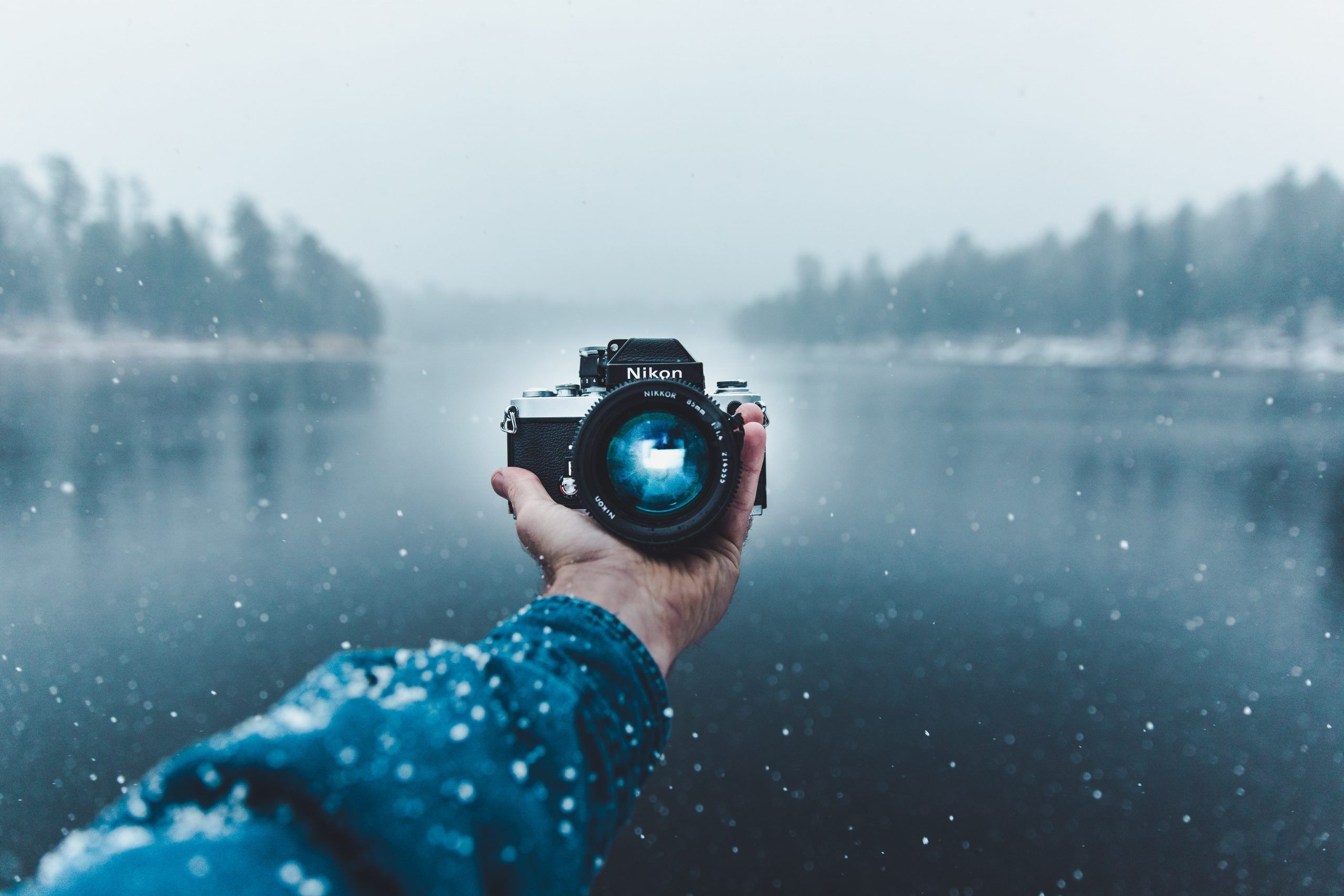
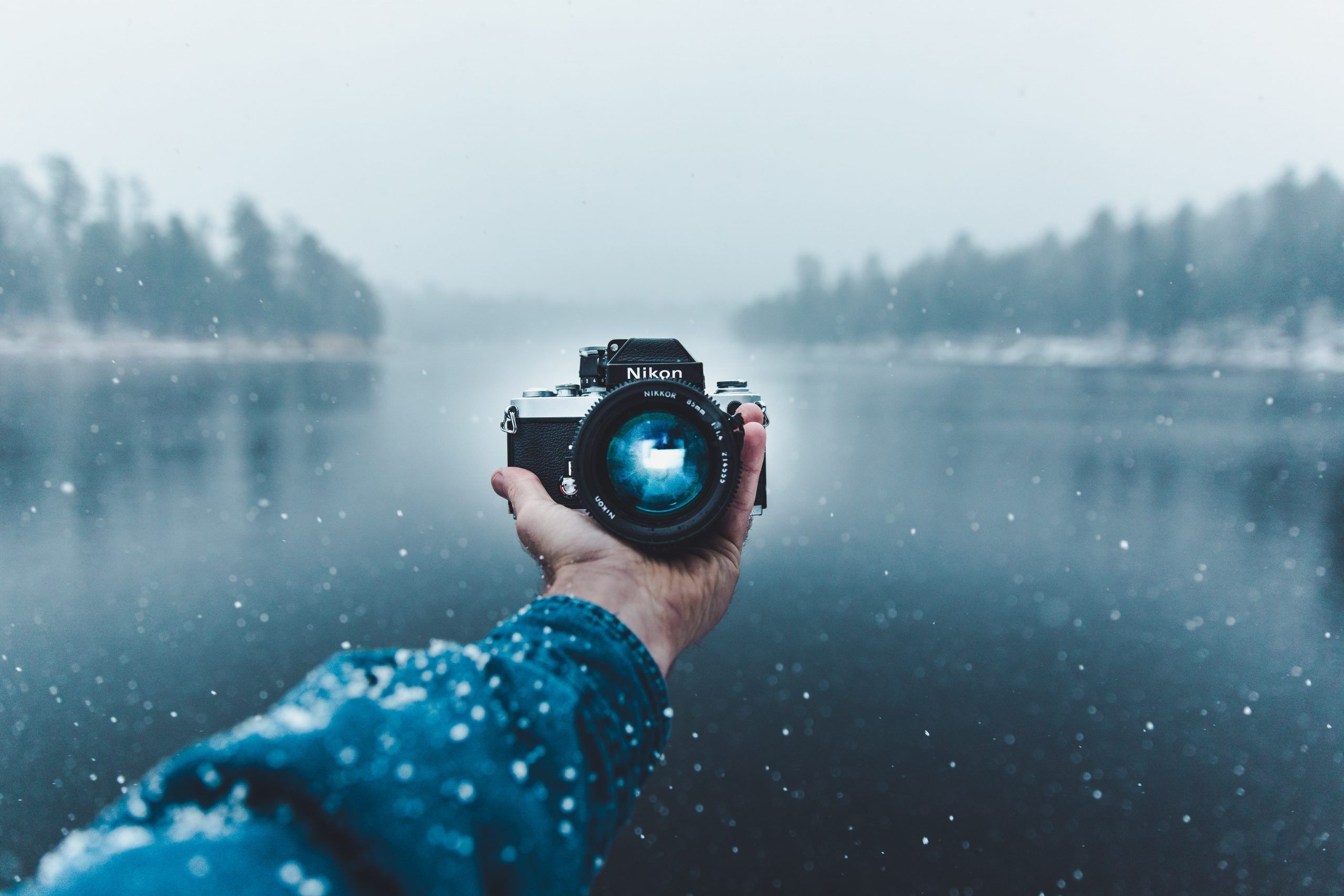
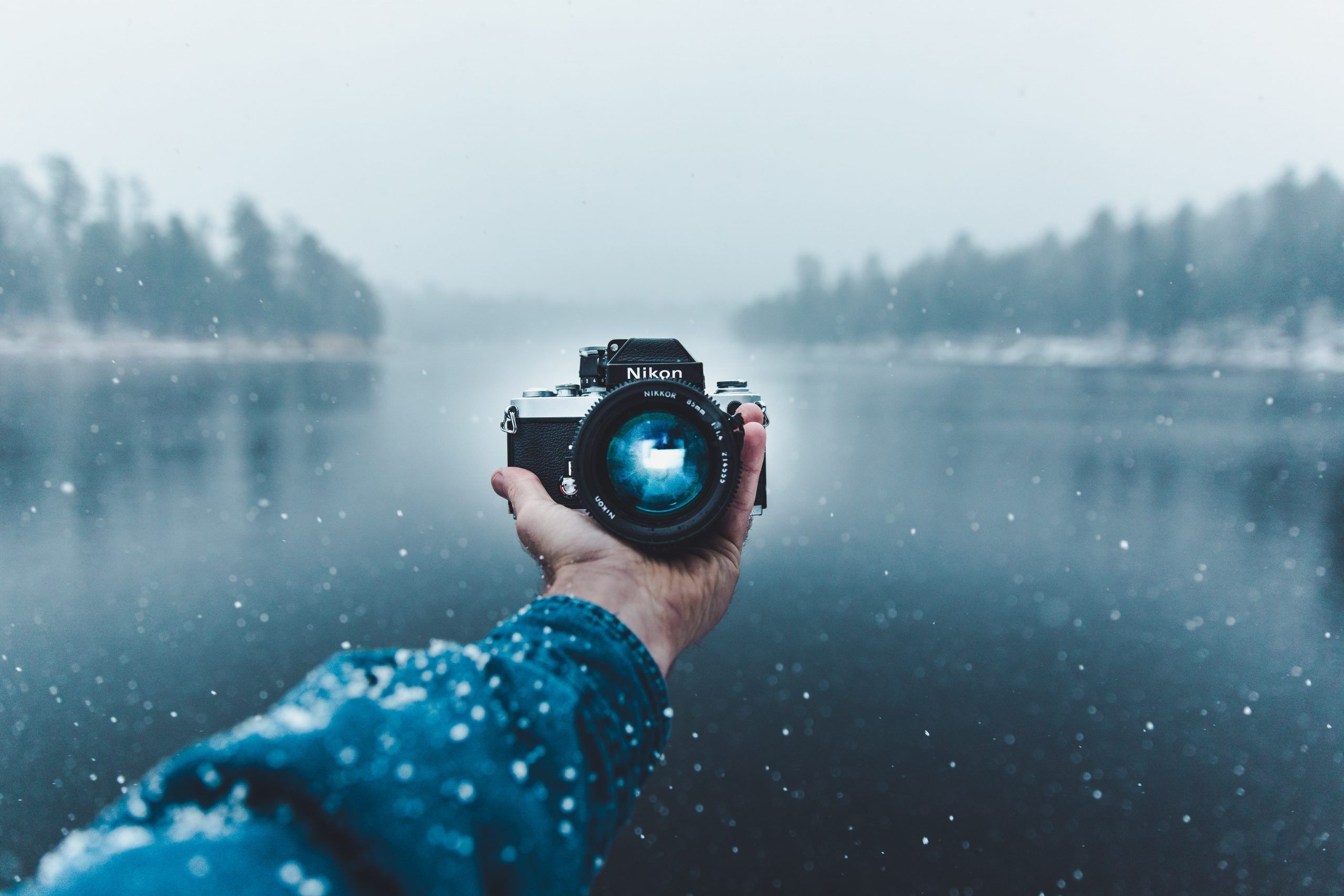
<div class="gallery-flex">
<div class="gallery-item">
<img src="image1.jpg" alt="Description">
</div>
<!-- More items -->
</div>
<style>
.gallery-flex {
display: flex;
flex-wrap: wrap;
gap: 15px;
}
.gallery-flex .gallery-item {
flex: 1 1 200px;
overflow: hidden;
}
.gallery-flex img {
width: 100%;
height: 200px;
object-fit: cover;
transition: transform 0.3s;
}
.gallery-flex .gallery-item:hover img {
transform: scale(1.05);
}
</style>
CSS Grid Gallery
A more structured gallery with consistent rows and columns using CSS Grid.

Nature

Architecture

Technology

Travel

Food

Art
<div class="gallery-grid">
<div class="gallery-item">
<img src="image1.jpg" alt="Description">
<div class="gallery-caption">Caption</div>
</div>
<!-- More items -->
</div>
<style>
.gallery-grid {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(250px, 1fr));
gap: 15px;
}
.gallery-grid img {
width: 100%;
height: 200px;
object-fit: cover;
transition: transform 0.3s;
}
.gallery-caption {
position: absolute;
bottom: 0;
background: rgba(0,0,0,0.7);
color: white;
transform: translateY(100%);
transition: transform 0.3s;
}
.gallery-item:hover .gallery-caption {
transform: translateY(0);
}
</style>